I have been learning Visual Basic .Net and have been trying to create many applications and I just wanted to put down what I have learned and this application would be a lot of help for those who are interested into programming or learning to code.
- How To Create A Calculator In Visual Basic
- Visual Basic Tip Calculator
- How To Create A Calculator In Visual Basic 2017
- Designing A Calculator In Visual Basic 2013 Part 1 - Visual ...
- How To Create A Calculator In Visual Basic Step By Step
- Visual Basic Calculator Program Code
The Calculator application is the most common application being asked in the examinations and also in the technical interview for job aspirants and I have found this one to be very useful and the code is also very simple and easy to learn and when actually starts creating it would be fun.
I am creating a VB 2008 change calculator as an assignment. The program is to use the amount paid - the amount due to calculate the total.(this is working fine). After that, it is to break that amount down into dollars, quarters, dimes, nickels, and pennies. How To Create A Calculator In VBNET In This VB.NET Tutorial we will See How To Make A Simple Windows Form Calculator Application With Swith To Do The Basic Operations (+, -, /,.) And Reset To Make Another Calc Using Visual Basic.Net In Visual Studio Editor. Introduction: Creating a Calculator Visual Studio C# This Instrucable will guide you through creating a basic calculator application in C# using Microsoft's Visual Studo development software. Visual Studio is a form of development software made by Microsoft to help developers create programs more easily.
I am putting down every thing in detail and in step by step form and also I would try to put in some images for easy understanding so that one would find its approach very easy and quick to learn. Also please find an attachment in pdf format of the code for calculator but a not of caution don't just copy paste the code but following step by step will actually help you to understand what is been done behind the code.
First of all you need to have Microsoft Visual Basic 6 or more or visual basic.net installed on your system. Open the application and it will display a start page if you do not have any other applications created. Now just follow the steps mentioned.
1. From the file menu select 'Standard EXE' from the new project menu (Visual basic 6). If working on Visual Basic. Net then from the File menu select New Project. You will see a blank form appearing on the screen.
2. Rename your project and your form by clicking on 'Project1' at the right hand side of the screen in the Solution Explorer window or in the project listing as per the version of the VB. Enter a new name in the 'Name' line of the Properties box, which should appear below the project listing by default. Press 'Enter' to accept the new name. Do the same for your form (a suggested form name is ‘Calculator'), making sure to enter a similar name in the 'Caption' property as well, which will change the text in the top bar of the form. Save the project in a new folder on your computer.
How To Create A Calculator In Visual Basic
3. Now you have a form displayed on the screen and also on the left side you would see the toolbox menu items. If you do not find it the go to the View Menu and click Tool bar and select the standard menu item. The tool box is displayed. Add the required buttons and a text box to the form.First add a text box in which the numbers are entered and also the results of calculations is displayed when the calculator appear. Select the Text Box button from the toolbar at the left side of the screen, and then drag with your mouse to the location you desire for the Text Box.
Once you've placed the Text Box you can change the size and location by dragging it to another location of the form or by dragging the handles (the small squares) along the border of the TextBox. Be sure to change the following lines in the Properties window, with the TextBox selected: '(Name)' = tbResult, 'Alignment' = 1- Right Justify, 'Data Format' = (click on the '...' button to select) Number, 'Locked' = True, and 'Text' = 0.
4. Select the Button icon on the toolbar and create the first button just like the way you created the Text Box. For quick reference use the Windows calculator in Standard view. You can view this by going to Programs > Accessories > Calculator as a base for your calculator layout.
We are not adding the 'MC', ‘MR', ‘MS' and ‘M+' buttons. For each button change the following properties using the '+' button as an example. Change the properties of Name as ‘btnPlus', Caption or Text as ‘+'. Do the same for the rest of the calculator buttons and save your work.
5. Now let's start adding some code to make the calculator work. Please note that your buttons and textbox should be named the same as the code listed here or else you will need to change the names to match your buttons and textbox to match this code. First we need to create a few variables for processing calculator input:
Dim aLeft As String, aRight As String, aOperator As String
Dim iLeft As Double, iRight As Double, iResult As Double
Dim bLeft As Boolean
Each calculation consists of four parts: a number to the left of the operator (aLeft, iLeft), an operator (aOperator), a number to the right of the operator (aRight, iRight), and a result (iResult). In order to track whether the user is entering the left or right number, we need to create a boolean variable, bLeft. If bLeft is true, the left side of the calculation is being entered; if bLeft is false, the right side is being entered.
6. Initialize the bLeft variable. We do that by creating a Form_Load subroutine, which you can either type as listed here or automatically create by double-clicking on any part of the form not covered by a button or textbox. Inside the function, we need to set bLeft to True, because the first number entered will be the left part:
Private Sub Form_Load()
bLeft = True
End Sub
7. Now we need to create a subroutine that will handle the clicking of any of the number buttons. This subroutine we be used for each of the number button and should be after the End Sub of the Form Load because we use identical code for each button, and using a subroutine means not having to repeat the same code ten times. Enter the following below the Form_Load subroutine's End Sub line:
Private Sub AddNumber(sNumber As String)
If bLeft Then
aLeft = aLeft + aNumber
tbResult.Text = aLeft
Else
aRight = aRight + aNumber
tbResult.Text = aRight
End If
End Sub
From the above we see that this function takes a string parameter, aNumber, which will contain the number the user has clicked on. If bLeft is true, this number is appended to the string that represents the number being entered, aLeft, and the textbox, tbResult, is updated to display the new number. If bLeft is false, the same operation is performed using aRight instead.
Finally, create a Click event function for each number that calls our AddNumber subroutine. You can do this easily by double-clicking each number button, which will create the subroutine structure for you. Then add the call to AddNumber, replacing the number in quotes with the number associated with the button. For the zero button, your code will look like this:
Private Sub btn0_Click()
AddNumber ('0')
End Sub
Likewise, for the one button, your code will look like this
Private Sub btn1_Click()
AddNumber ('1')
End Sub
Like wise create the subroutine for all other numbers.
8. To handle the operators plus, minus, times, and divide. We will do this step, creating a subroutine that is called in the Click events for the operator buttons. The subroutine will look like the following:
Private Sub AddOperator(sNewOperator As String)
If bLeft Then
aOperator = sNewOperator
bLeft = False
Else
btnEquals_Click (this should be used only in Visual Basic 6. If the same is there in the VB.net it will throw error. Make a note of it)
aOperator = sNewOperator
aRight = '
bLeft = False
End If
End Sub
If bLeft is true, meaning the user has just entered the left part of the calculation, this subroutine sets the aOperator variable we created in step 5 to equal the operator entered, which is passed to AddOperator as the string sNewOperator. The second step is to set bLeft to False, because the entry of an operator means the user is done entering the left side of the equation.
In order to handle entries that string multiple operators together, such as 9 * 3 * 2 * 6, we need to also check whether bLeft is false, meaning the user has entered an operator where we were expecting an equals. First we call the Click event for the equals button (described in the next step), which does the calculation and sets tbResult to the result of what has already been entered. Then we clear aRight so the user can enter the next number, and set bLeft to False so the program knows we are entering the right hand side of the calculation next.
Add an AddOperator call to the Click event of each operator button, using the same method as we used in step 7 to create the Click events for the number buttons. Your code for the plus button will look like this:
Private Sub btnPlus_Click()
AddOperator ('+')
End Sub
Likewise, the code for the minus button will look like this:
Private Sub btnMinus_Click()
AddOperator ('-')
End Sub
9. Create the Click event for the equals button, which is the most complex code in this program. Create the subroutine structure like you did for the other buttons, by double-clicking the equals button on your form.
Here in this subroutine we will use the case syntax because based on the button click the code should run. So the case statement will select the button clicked like plus, minus, divide, multiply etc and will perform the condition mentioned for that particular case. So the advantage of the case syntax is that it will directly go to the required condition. Your subroutine will look like this when you've entered the code:
Private Sub btnEquals_Click()
If aLeft <> ' And aRight = ' And aOperator <> ' Then
aRight = aLeft
End If
If sLeft <> ' And aRight <> ' And aOperator <> ' Then
iLeft = aLeft
iRight = aRight
Select Case sOperator
Case '+'
iResult = iLeft + iRight
Case '-'
iResult = iLeft - iRight
Case '/'
iResult = iLeft / iRight
Case '*'
iResult = iLeft * iRight
End Select
tbResult.Text = iResult
aLeft = iResult
aRight = '
bLeft = True
End If
End Sub
The first three lines of code check to see if both sides of the calculation have been entered along with an operator. If only the left side and an operator are entered, the value of the left side is copied to the right, so we can mimic the standard calculator behavior for handling an entry like 9 * =, which multiplies 9 by itself to get a result of 81.
The rest of the code will run only if left, right, and operator are entered, and starts out by copying the strings of numbers into our iLeft and iRight Double-typed variables, which can do the actual calculations. The Select Case statement allows us to run different code depending on which operator was entered, and performs the actual calculation, placing the result in iResult. Finally, we update the textbox with the result, copy the result into aLeft, reset aRight, and set bLeft = True.
These last lines allow us to take the result of the calculation and use it to perform another calculation.
10. Now we will look at the last three operation buttons: sqrt, %, and 1/x. For the Click event of the square root button, your code will look like this:
Private Sub btnSqrt_Click()
If aLeft <> ' Then
iLeft = aLeft
Else
iLeft = 0
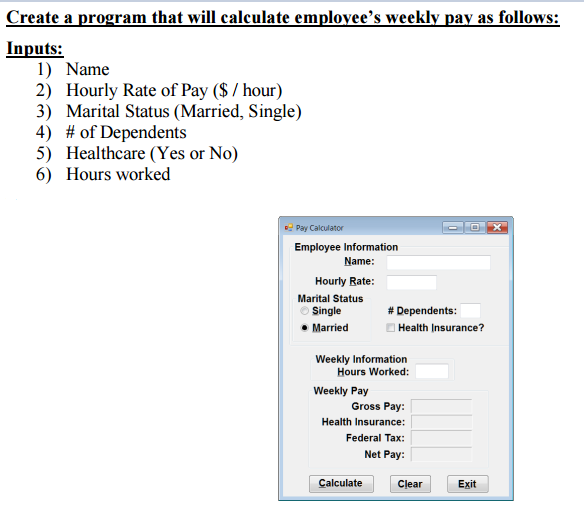
End If
If aRight <> ' Then
iRight = aRight
Else
iRight = 0
End If
If bLeft Then
iLeft = Math.Sqr(iLeft)
tbResult.Text = iLeft
Else
iRight = Math.Sqr(iLeft)
tbResult.Text = iRight
End If
If iLeft <> 0 Then
aLeft = iLeft
Else
aLeft = '
End If
If iRight <> 0 Then
aRight = iRight
Else
aRight = '
End If
End Sub
A point to be noted is that when the square root function is run then in the iLeft = Math.Sqr(iLeft), iRight = Math.Sqr(iLeft) lines sqr to be changed to sqrt if using VB.net.
The first 11 lines of code make sure that if we don't have a value entered for either side of the equation, we substitute zero instead of trying to copy an empty string into iLeft or iRight, which will generate an error. The middle lines perform the square root function on the current part of the calculation, either left or right. Finally, we reverse the checks we did in the beginning so that a zero is copied as an empty string back into aLeft and aRight.
For the percent button, the code is similar, with one exception: the percent operation can only be performed if both left and right sides are entered.
Private Sub btnPercent_Click()
If Not bLeft Then
If aRight <> ' Then
iRight = aRight
Else
iRight = 0
End If
iRight = iRight * (iLeft / 100)
tbResult.Text = iRight
If iRight <> 0 Then
aRight = iRight
Else
aRight = '
End If
End If
End Sub
Lastly, the 1/x, or fraction, Click event, which is very similar to the code above:
Private Sub btnFraction_Click()
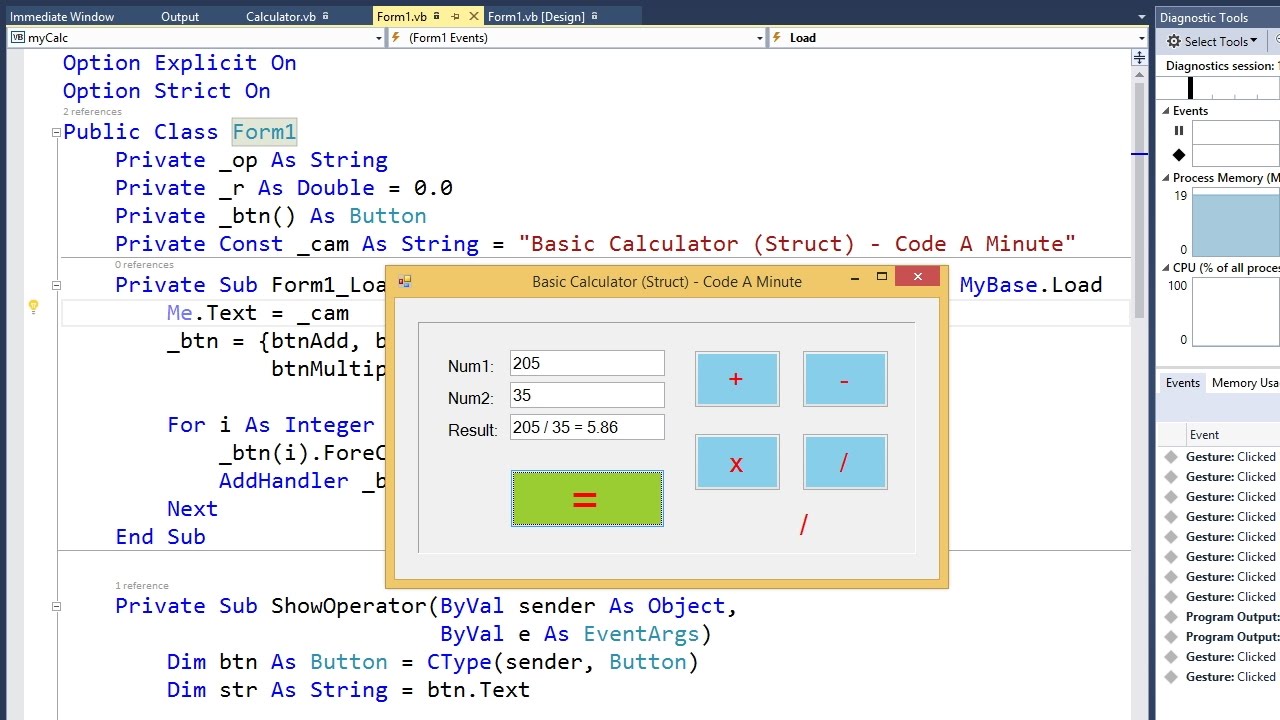
If aLeft <> ' Then
iLeft = aLeft
Else
iLeft = 0
End If
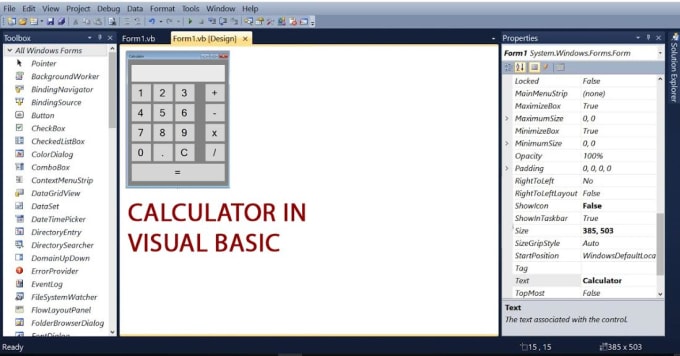
If aRight <> ' Then
iRight = aRight
Else
iRight = 0
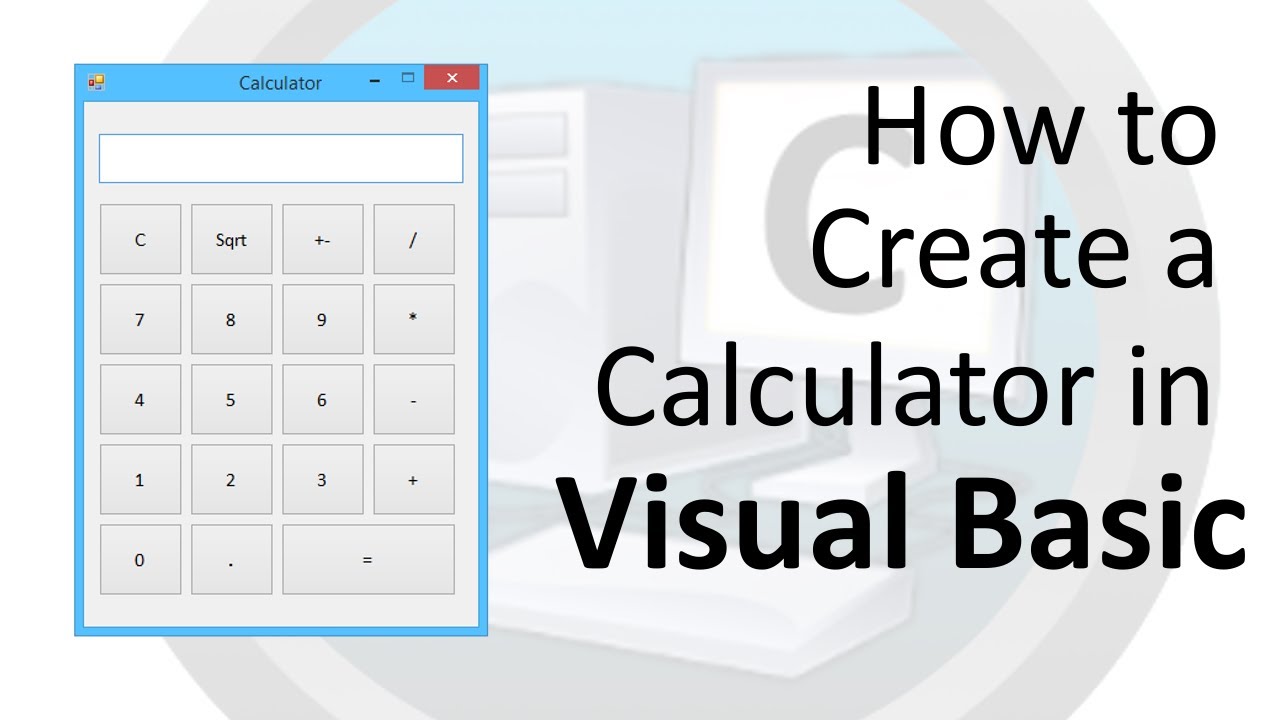
If bLeft Then
iLeft = 1 / iLeft
tbResult.Text = iLeft
Else
iRight = 1 / iRight
tbResult.Text = iRight
End If
If iLeft <> 0 Then
aLeft = iLeft
Else
aLeft = '
End If
Visual Basic Tip Calculator
If iRight <> 0 Then
aRight = iRight
Else
aRight = '
End If
End Sub
11. Lets add some code to handle the C and CE buttons. C clears all input to the calculator, whereas CE only clears the number currently being entered.
Private Sub btnC_Click()
aLeft = '
aRight = '
aOperator = '
tbResult.Text = '0'
bLeft = True
End Sub
Private Sub btnCE_Click()
If bLeft Then
aLeft = '
Else
aRight = '
End If
tbResult.Text = '0'
How To Create A Calculator In Visual Basic 2017
End Sub
12. Here we are now we are ready with the calculator and can run your calculator program and do any calculation you wish. Now by putting in extra effort and some thinking this calculator can be easily expanded to handle more operations, more complex calculations or even to be a scientific calculator.
“first of open a new window form
then insert a 8 label , 2 textbox and 6 buttons
change the all tools text properties like and arrange all buttons and textbox.
then double click one the form and create a variable.
then double click on the buttons and write a coding.
then create a menu from a toolbox and write a text for a menu
then save a program and run
-~-–~-~~-~-
The Coding Bus
How to link one page to another page in HTML
https://youtu.be/GmzUr4Tdeb0
How to create a simple Home Design program in java Applet
https://youtu.be/oLtG5vNI7WA
Difference between Core JAVA VS Advanced JAVA
https://youtu.be/iR3uxTlE7nw
How to change Form background color in Runtime in VB.net
https://youtu.be/Ewd9Ow_qmsE
How to create the Rainbow in Java Applet
https://youtu.be/gjGq9zKTZpg
How to create a game in visual basic(Ant hit game in vb)
https://youtu.be/MdhwSYo8qNk
https://youtu.be/y3Rq-w5UQzY
Marksheet program in C language
The Coding Bus
qwertyuiopasdfghjklzxcvbnm
Designing A Calculator In Visual Basic 2013 Part 1 - Visual ...
How to install wordpress in xampp step by step
https://www.youtube.com/watch?v=YdwMhXX-FLE
How to create menu and submenu in wordpress
https://www.youtube.com/watch?v=PACC3farNPY
https://www.youtube.com/watch?v=8kG3JTbGAbw
How to install wordpress theme with demo data free
How to change footer copyright in wordpress
https://www.youtube.com/watch?v=3oIkPWYXyyQ
How to change footer widget in wordpress
https://www.youtube.com/watch?v=1HbnBbZX9tA
How to backup & restore your wordpress website in 3 minutes free 2018
https://www.youtube.com/watch?v=pSr5w4U36c8
How to use the revolution slider plugin with Button Link – full tutorial 2018
https://www.youtube.com/watch?v=KMBFxOlObx4
https://www.youtube.com/watch?v=z5jbofGMOHM
How to add new user role in wordpress
How To Add YouTube Video To Your WordPress Website 2018
https://www.youtube.com/watch?v=jCWQ7oA2bBA
How to add contact form 7 in wordpress page
https://www.youtube.com/watch?v=E9U27FOPNyo
How to change favicon in wordpress theme
https://www.youtube.com/watch?v=uvczOIqdVYk
How to use tubebuddy on youtube – 2018 full tutorial
https://www.youtube.com/watch?v=N1zpn3sT-2o
How to add additional css in wordpress 2018
https://www.youtube.com/watch?v=2L7lHf_0C-E
qwertyuiopasdfghjklzxcvbnm
-~-–~-~~-~- How to create an app for free using your mobile phone 2019
https://youtu.be/VxmK-giIyGA
How to delete history in google chrome 2019 android
https://youtu.be/2YJqas7Dwno
how to create camera app in mit app inventor 2
https://youtu.be/o-bYys8v9g4
how to redirect http to https in WordPress website
https://youtu.be/dLrsJboGzRg
How to install wordpress in xampp step by step
https://youtu.be/YdwMhXX-FLE?list=PL7sbjUYIdF3Ueka8gN7sNX8VoBKoBYSFJ
How To Create A Calculator In Visual Basic Step By Step
How to add youtube video to wordpress website
https://youtu.be/YdwMhXX-FLE?list=PL7sbjUYIdF3Ueka8gN7sNX8VoBKoBYSFJ
how to create child theme in WordPress 2019
https://youtu.be/zM-CT9wFtw8?list=PL7sbjUYIdF3Ueka8gN7sNX8VoBKoBYSFJ
Visual Basic Calculator Program Code
sdfhfdghjhjlqwerqwes werte qwexvnkl #USA lsdkfjsdlkfjd dfkajsdlfj lkdjsflkdjfdls visual studio tutorial
visual studio code tutorial
visual studio c# tutorial
visual basic programming tutorial
visual studio for beginners
visual c++ tutorial
visual studio 2015 tutorial for beginners
visual studio 2017 tutorial
xamarin tutorial visual studio 2017
visual c# tutorial
visual studio c++ tutorial
visual basic codes for beginners
visual studio introduction
visual studio code tutorial pdf
visual studio android app tutorial
visual basic 2010 tutorial pdf
visual basic 2010 tutorial
c# web application tutorial visual studio 2017
visual studio 2017 android app tutorial
visual basic 2017 tutorial
visual studio 2017 python tutorial
microsoft visual studio tutorial
visual studio team services tutorial
visual studio 2017 tutorials for beginners pdf visual studio tutorial
visual studio code tutorial
visual studio c# tutorial
visual basic programming tutorial
visual studio for beginners
visual c++ tutorial
visual studio 2015 tutorial for beginners
visual studio 2017 tutorial
xamarin tutorial visual studio 2017
visual c# tutorial
visual studio c++ tutorial
visual basic codes for beginners
visual studio introduction
visual studio code tutorial pdf The Coding Bus #unitedstates
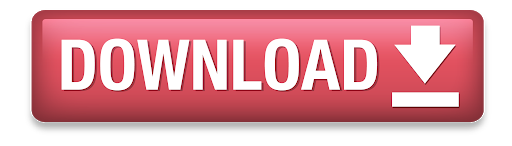